Android CameraX was designed to make camera development easier. As CameraX was being developed, camera application developers have shared their passion and enthusiasm with us, and many great ideas have been built into the current API. One great example is the CameraX Extensions API. We’ve recently rearchitected these extensions with input from the developer community, and now with the new ExtensionsManager, you can use these extensions with as little as two lines of code! This post covers how to use the Extensions API in your app.
CameraX Extensions
Android devices contain powerful cameras, with manufacturers devoting a lot of effort to build many cutting-edge features, or special effects, into these camera devices. These powerful features used to be accessible only to the device’s native camera applications. With the CameraX Extensions API, third-party developers can now access these powerful camera features through a common, simple interface.
From our partners:
CameraX Extensions scope
Version 1.0.0 of the CameraX Extensions includes some of the most common built-in camera special effects:
- BOKEH: making foreground people sharper when photos are taken in portrait mode.
- HDR: taking photos with different Auto Exposure (AE) settings to generate the best result.
- NIGHT: getting the best still images under low-light situations, typically at night time.
- FACE RETOUCH: retouching face skin tone, geometry, and so on when taking still images.
- AUTO: automatically adjusting the final image based on the surrounding scenery.
Let’s look at a couple of example photos taken with and without enabling special effects via CameraX Extension API on an Android phone.
BOKEH mode example

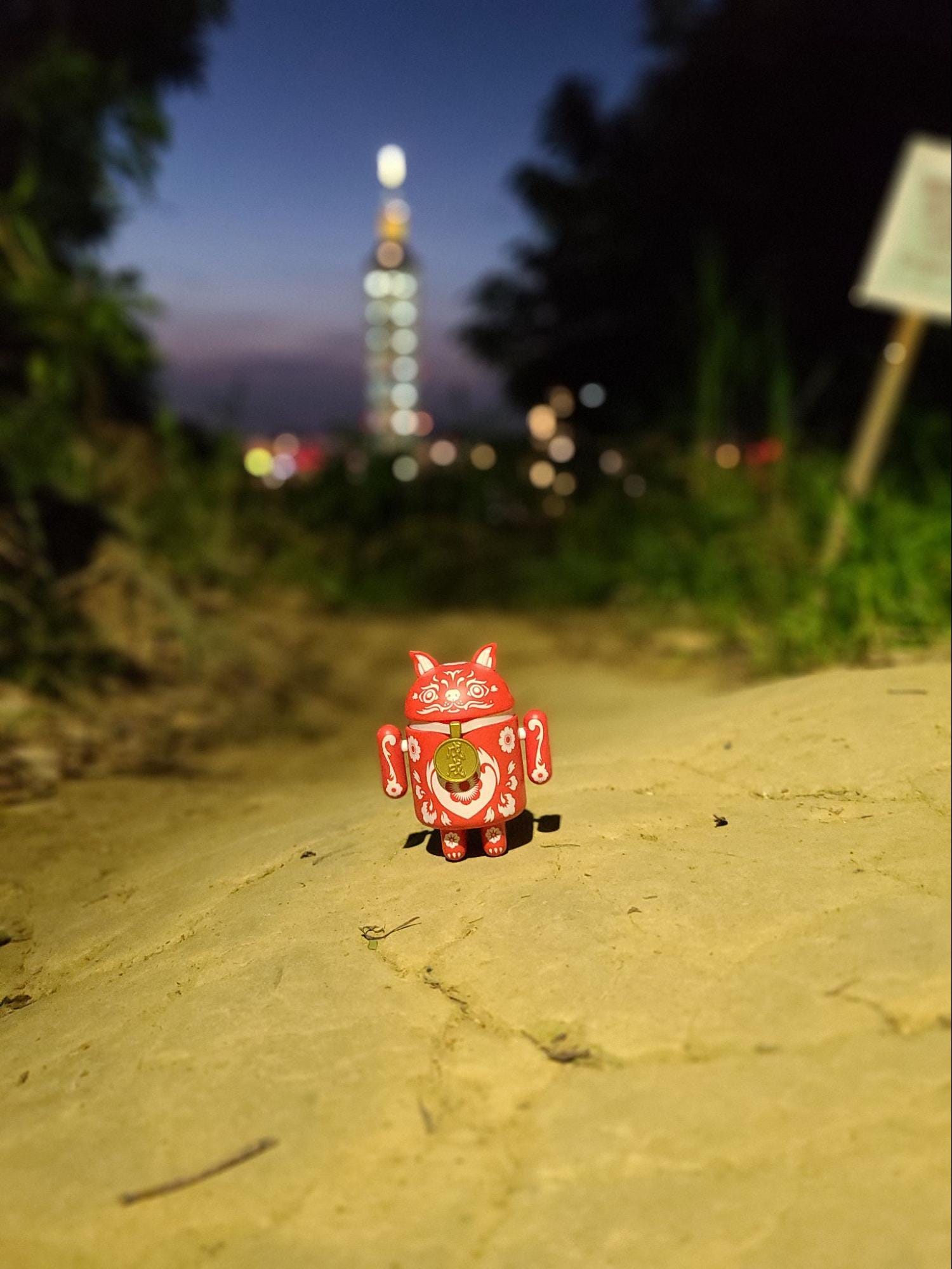
HDR mode example
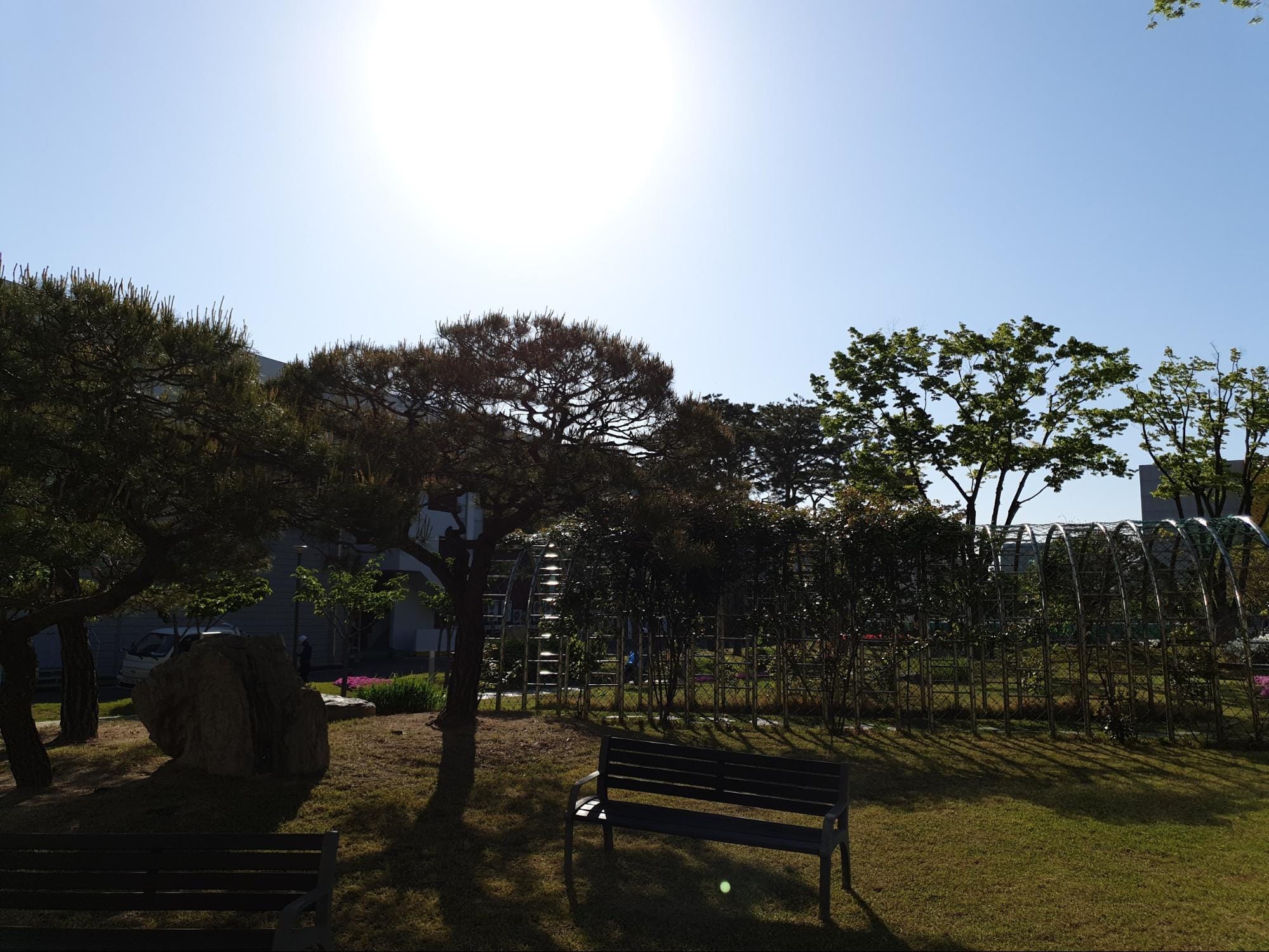
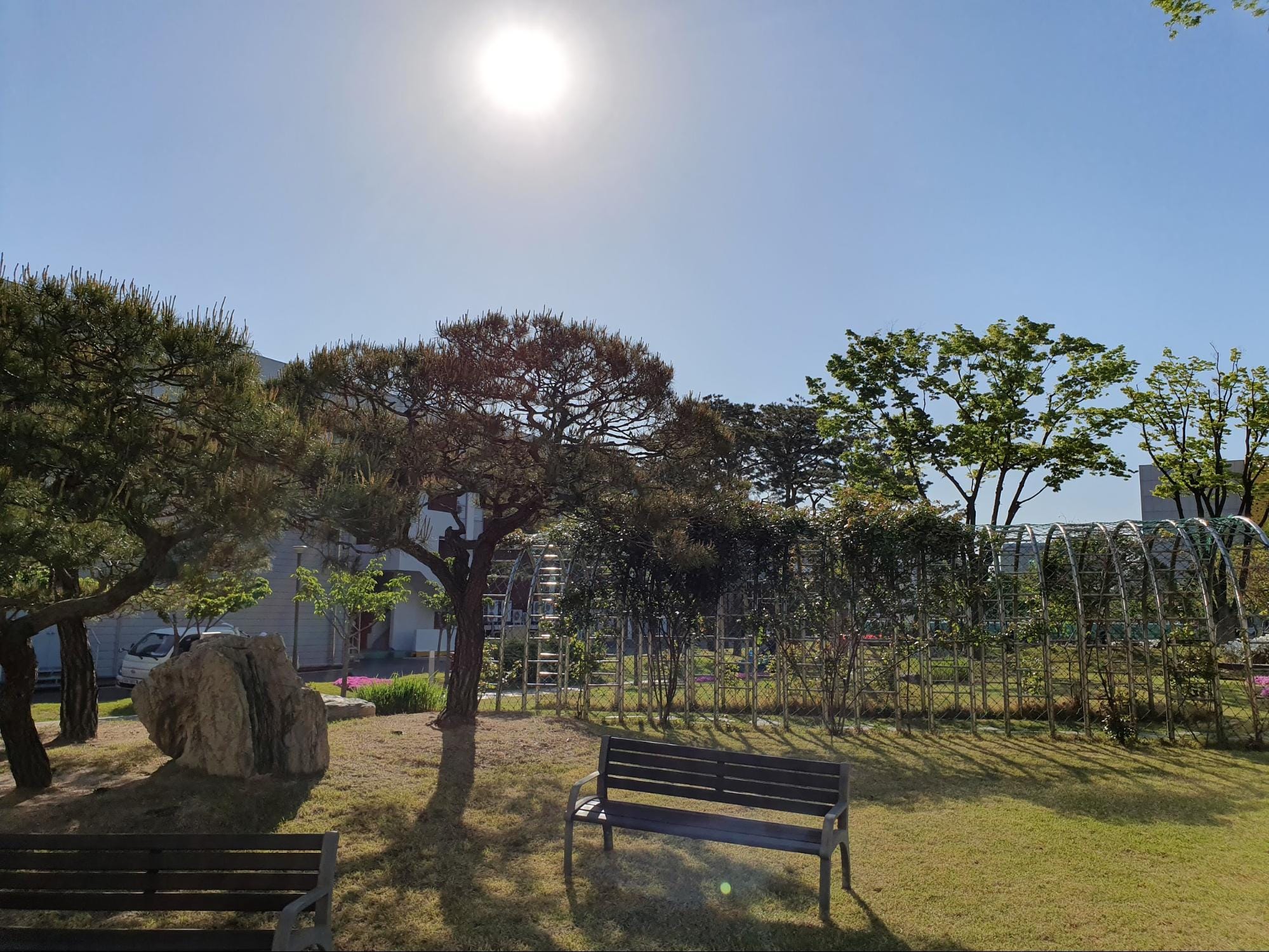
NIGHT mode example
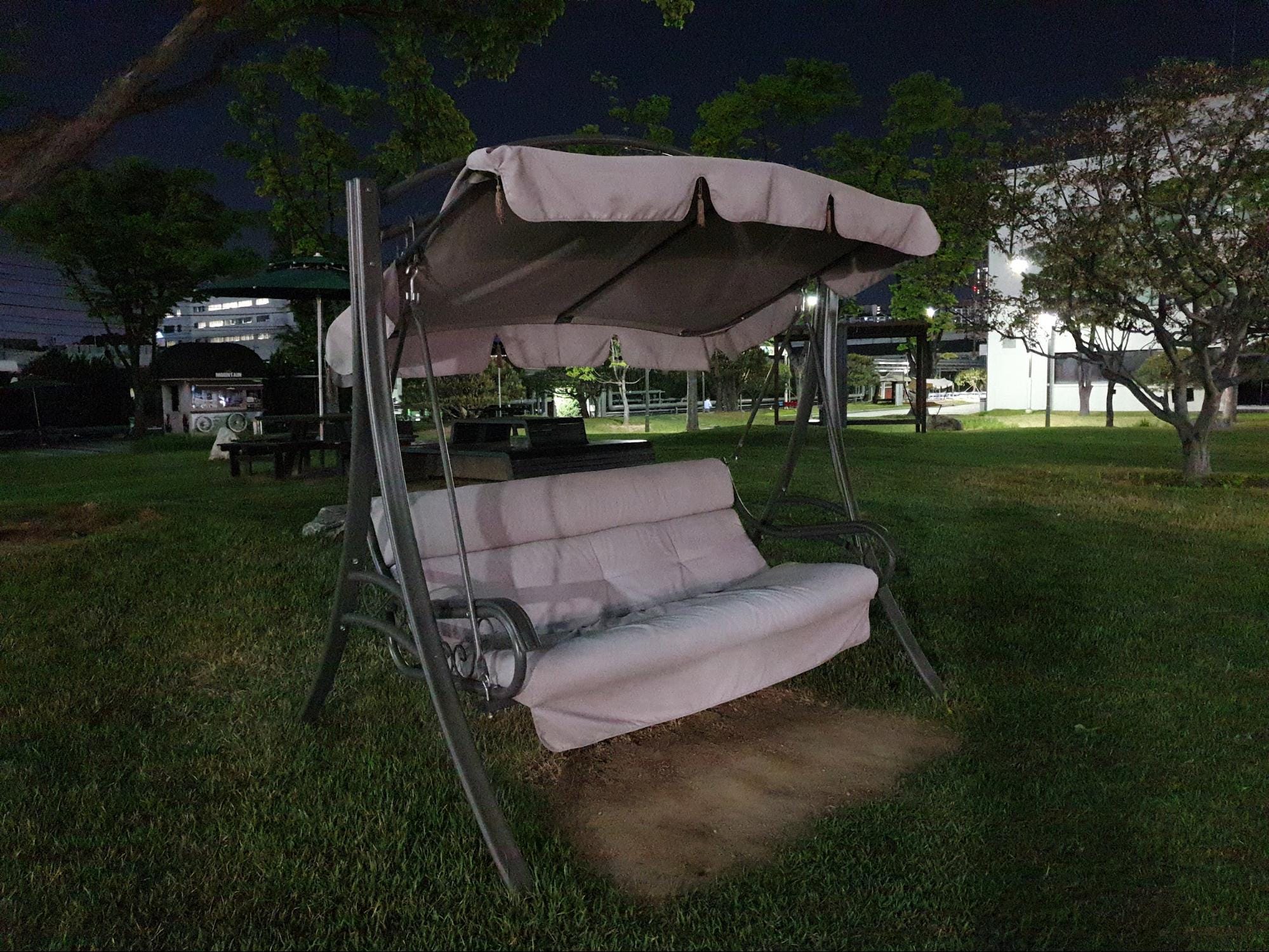
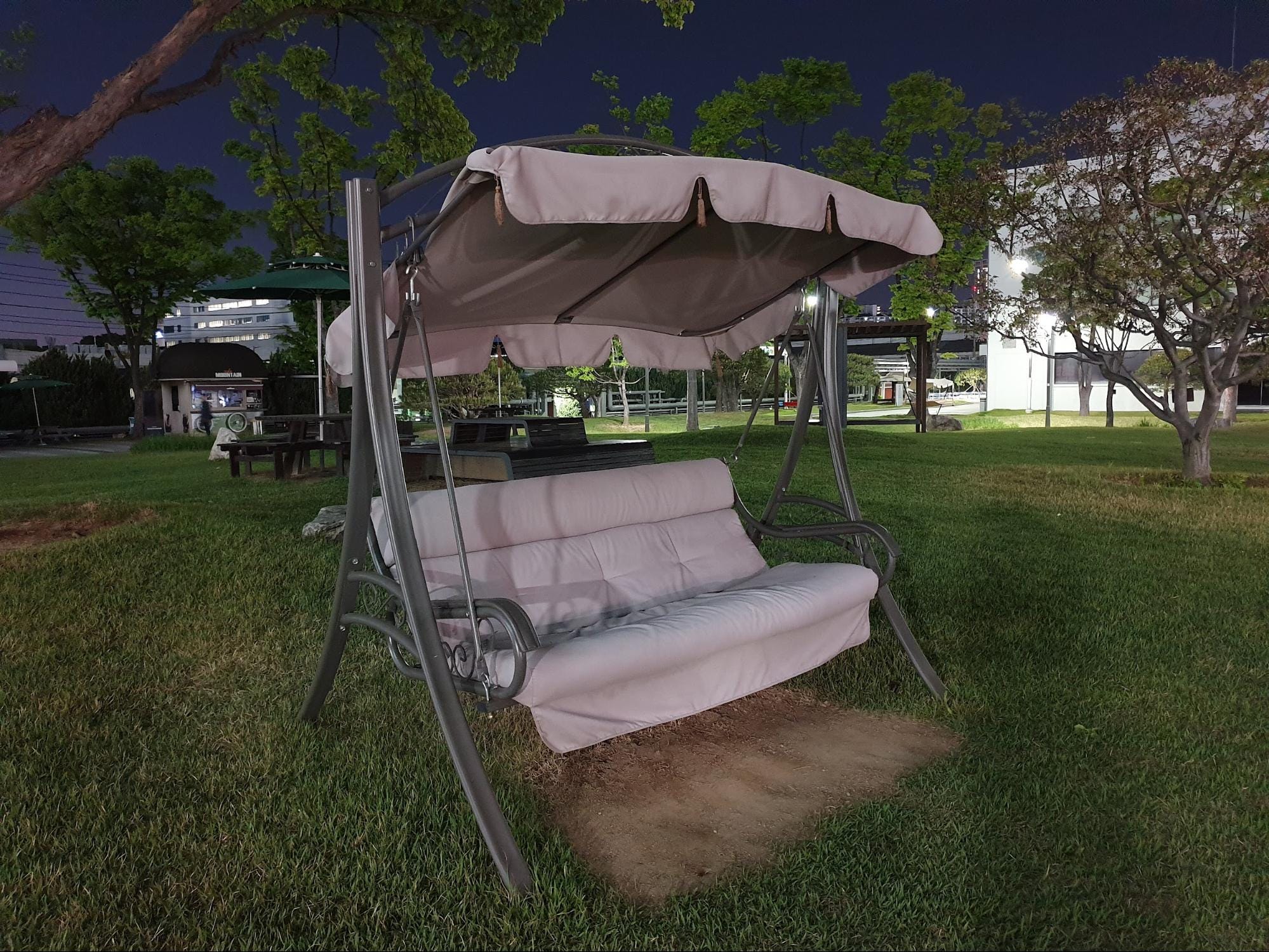
The visual difference is clear. You can use the CameraX Extensions API to make these images in your own applications.
Now let’s see how to integrate the CameraX API into your application.
Extensions API
In an existing CameraX app, you can add CameraX Extensions by first including the camera-extensions
Jetpack library:
dependencies { // CameraX core libraries which version matches the extensions library implementation 'androidx.camera:camera-core:1.1.0-alpha08' implementation 'androidx.camera:camera-camera2:1.1.0-alpha08' implementation 'androidx.camera:camera-lifecycle:1.1.0-alpha08' // CameraX Extensions library implementation 'androidx.camera:camera-extensions:1.0.0-alpha28' // other dependencies implementation('androidx.concurrent:concurrent-futures-ktx:1.1.0') … }
Next, integrate the extensions with the following steps:
- Acquire the ExtensionsManager instance.
- Check whether the target device supports the intended extension mode.
- Get an extension-enabled
CameraSelector
. - Call bindToLifecycle using the extension-enabled
CameraSelector
.
Acquire the ExtensionsManager instance
The first step is to get an ExtensionsManager instance with its getInstance(Context) API. This API returns a ListenableFuture
where we can use await()
to get the result in the Kotlin suspend function to avoid blocking the main thread. (Note: using await()
on ListenableFuture
requires the androidx.concurrent:concurrent-futures-ktx: 1.1.0
dependency.)
// Creates the extensions manager (with Jetpack Concurrent library) val extensionsManager = ExtensionsManager.getInstance(context).await()
ExtensionsManager allows you to determine device support and get an extension-enabled CameraSelector
for a specific extension mode. Note the following:
- ExtensionsManager is a process-scoped global resource: only a single ExtensionsManager instance exists within a process.
- ExtensionsManager always exists: CameraX provides a functioning ExtensionsManager instance regardless of whether the underneath device supports any extension(s).
Check the Extension Mode Availability
With the ExtensionsManager, check for the extension availability using the isExtensionAvailable(CameraProvider, CameraSelector, int) function: it returns true
If any camera filtered by the CameraSelector
on the device supports the queried extension, false
otherwise.
// Get the list of camera devices to check for extension availability val cameraSelector = CameraSelector.DEFAULT_BACK_CAMERA // Checks whether bokeh is supported if (extensionsManager.isExtensionAvailable( cameraProvider, cameraSelector, ExtensionMode.BOKEH )) { ... }
Get an extension-enabled CameraSelector
Once you know that the extension mode is supported on the device, retrieve an extension-enabled CameraSelector
with the getExtensionEnabledCameraSelector(CameraProvider, CameraSelector, int) function. The returned extension-enabled CameraSelector
contains all the details about the specified extension mode.
val bokehCameraSelector = extensionsManager .getExtensionEnabledCameraSelector( cameraProvider, cameraSelector, ExtensionMode.BOKEH)
Call bindToLifecycle() using the extension-enabled CameraSelector
The final step is to bind your use cases with the extension-enabled CameraSelector
using bindToLifecycle(). The extension-enabled CameraSelector
can be used just like a normal CameraSelector
, such as with DEFAULT_BACK_CAMERA or DEFAULT_FRONT_CAMERA. CameraX enables the specified extension mode directly on the camera when binding use cases with the extension-enabled CameraSelector
. For example, the extension effect is applied onto the preview when Preview
is bound, or onto the images captured by the bound ImageCapture
.
// Binds use case with the bokeh enabled camera selector val imageCapture = ImageCapture.Builder().build() val preview = Preview.Builder().build() cameraProvider.bindToLifecycle( lifecycleOwner, bokehCameraSelector, imageCapture, preview )
Extensions API usage sample code
The full code for this extensions API example looks like the following:
fun onCreate() { lifecycleScope.launch { // Creates the camera provider val cameraProvider = ProcessCameraProvider.getInstance(context).await() // Creates the extensions manager (with Jetpack Concurrent library) val extensionsManager = ExtensionsManager.getInstance(context).await() // Get the list of camera devices to check for extension availability val cameraSelector = CameraSelector.DEFAULT_BACK_CAMERA // Checks whether bokeh is supported if (extensionsManager.isExtensionAvailable( cameraProvider, cameraSelector, ExtensionMode.BOKEH )) { // Unbinds all use cases before enabling different extension modes. cameraProvider.unbindAll() // Gets the bokeh enabled camera selector val bokehCameraSelector = extensionsManager .getExtensionEnabledCameraSelector( cameraProvider, cameraSelector, ExtensionMode.BOKEH ) // Binds use case with the bokeh enabled camera selector val imageCapture = ImageCapture.Builder().build() val preview = Preview.Builder().build() cameraProvider.bindToLifecycle( lifecycleOwner, bokehCameraSelector, imageCapture, preview ) } } }
Extensions API dependencies on core modules
The CameraX Extensions API is implemented in the camera-extensions
library and has a dependency on the CameraX core modules (core,camera2,lifecycle
). When using CameraX Extensions, be sure to use the version from the same release package as the CameraX core modules that you’re using. For example, to use the camera-extensions:1.0.0-alpha28
, you must also include version 1.0.0-alpha08
for the camera-lifecycle, camera-core, camera-camera2
into application’s dependency list, as they were released in the same package on August 18, 2021.
Extensions supported devices
To use the CameraX extensions API, device manufacturers need to implement CameraX Vendor Extensions interfaces. You can find a partial list of the CameraX Extensions API supported devices on the CameraX devices page. Note that this is not an exhaustive list. If your device is listed but the availability checks return false, you might need to update your device to the latest ROM version from your manufacturer.
In addition to the supported devices list, starting with Android 12, you can also check the Android property ro.camerax.extensions.enabled
to determine whether the device supports CameraX Extensions.
Legacy Extensions API removal
CameraX released a legacy Extensions API in August 2019. This legacy Extensions API provided extender classes which are needed to apply extensions-related configuration onto each Preview
and ImageCapture
use case. The legacy extender design might cause the developers to miss enabling extension mode on either Preview
or ImageCapture
and could cause unintended behavior.
The new CameraX Extensions library was introduced in 1.0.0-alpha26
. The newer Extensions API switches extension binding from use cases to the target camera and are much easier to use. Be sure to migrate to take advantage of the new Extensions API.
We especially appreciate our awesome Android camera developers and device manufacturers that help to make the CameraX Extensions API happen! If you’d like to stay in touch with the latest CameraX development, join the Android CameraX Discussion Group.
Other references
By Charcoal Chen
Source Medium
For enquiries, product placements, sponsorships, and collaborations, connect with us at [email protected]. We'd love to hear from you!
Our humans need coffee too! Your support is highly appreciated, thank you!